CSS
Images
CSS allows the user to style the image in different ways.
Text on Image
Code
<!doctype html>
<html>
<head>
<title>...</title>
<style type="text/css">
img {
position: absolute;
left: 0px;
top: 0px;
z-index: -1;
}
</style>
</head>
<body>
<img src="myimage.png">
<p>Tutorials for Web Programming</p>
</body>
</html>
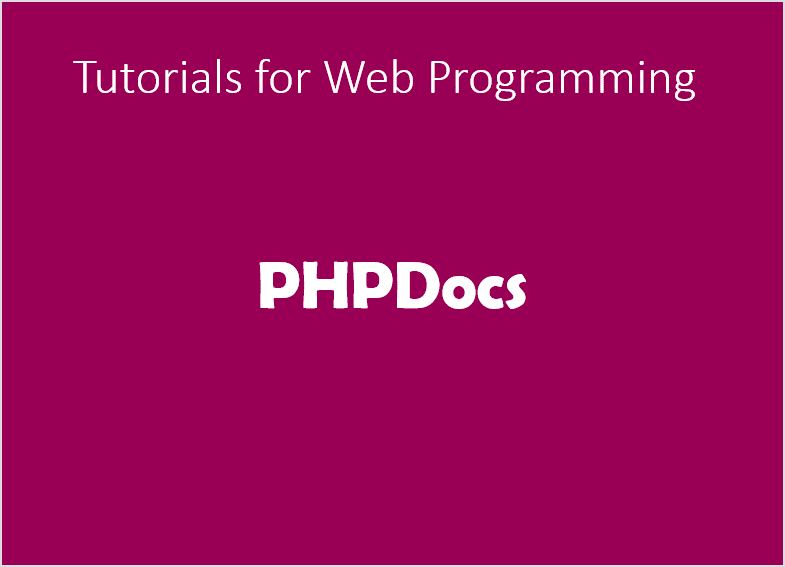
Rounded Image
Code
<!doctype html>
<html>
<head>
<title>...</title>
<style type="text/css">
img{
width: 56%;
border-radius: 55%;
height: 50%;
}
</style>
</head>
<body>
<img src="myimage.png">
</body>
</html>

Polaroid Image
Code
<!doctype html>
<html>
<head>
<title>...</title>
<style type="text/css">
div.polaroid {
width: 80%;
margin-bottom: 25px;
background-color: white;
box-shadow: 0 4px 8px 0 rgba(0, 0, 0, 0.2), 0 6px 20px 0 rgba(0, 0, 0, 0.19);
}
div.caption {
text-align: center;
padding: 10px 20px;
}
</style>
</head>
<body>
<div class="polaroid">
<img src="img_5terre.jpg" alt="5 Terre" style="width:100%">
<div class="container">
<p>Cinque Terre</p>
</div>
</div>
</body>
</html>

Image Transparency
Code
<!doctype html>
<html>
<head>
<title>...</title>
<style type="text/css">
img {
opacity: 0.5;
}
</style>
</head>
<body>
<img src="myimage.png">
</body>
</html>
